Discover ways to create a blockchain explorer utilizing JavaScript (NodeJS and NextJS) in simply over one hour by following alongside on this article. The explorer will probably be an Etherscan clone that may fetch parsed on-chain information utilizing the Moralis Web3 Information API. As we progress, you’ll see how straightforward it’s to work with this API to cowl our blockchain explorer’s blockchain-related backend features. Now, earlier than we transfer into the tutorial part, let’s have a look at the endpoints we are going to use:
The Token API’s getTokenPrice endpoint to get the ERC-20 token worth: const response = await Moralis.EvmApi.token.getTokenPrice({
handle: “0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2”,
chain: “0x1”,
}); The Block API’s getDateToBlock and getBlock endpoints to get the block by date and hash:const latestBlock = await Moralis.EvmApi.block.getDateToBlock({
date: Date.now(),
chain: “0x1”,
});const previousBlockNrs = await Moralis.EvmApi.block.getBlock({
chain: “0x1”,
blockNumberOrHash: blockNrOrParentHash,
}); The Transaction API’s getWalletTransactionsVerbose endpoint to get decoded transactions by pockets:const response =
await Moralis.EvmApi.transaction.getWalletTransactionsVerbose({
handle: question.handle,
chain,
});
Odds are you have already got some expertise with frontend improvement, so we determined to deal with the backend features of a blockchain explorer. That mentioned, we are going to be sure you discover ways to implement the above code snippets appropriately. So far as the frontend goes, you possibly can depend on the video on the prime because it explores that half in additional element.
So, when you want to create a blockchain explorer following our lead, make certain to enroll with Moralis now! You can begin with a free plan, because it offers you entry to all Moralis Web3 API endpoints. Nevertheless, when you anticipate your dapps to obtain a bigger quantity of customers, the Moralis Professional plan is the go-to alternative.
Overview
Blockchain explorers, often known as “block explorers”, allow devs and different blockchain customers to discover on-chain information. We’ve beforehand touched on this matter as we created a easy information exhibiting methods to simply construct a block explorer. That article can be a terrific place to be taught what blockchain explorers are and their advantages. Nevertheless, this text is extra of an entire, detailed information on methods to create an Etherscan-grade blockchain explorer.
All through the next sections, we are going to deal with exhibiting you methods to create a blockchain explorer backend with NodeJS and Moralis. We’ll additionally do a fast demo, serving to you resolve whether or not or not you need to construct the frontend half and create your personal occasion of our Etherscan clone. Beneath the backend tutorial and our demo, you can too discover the reply to the “what’s a blockchain explorer?” query.
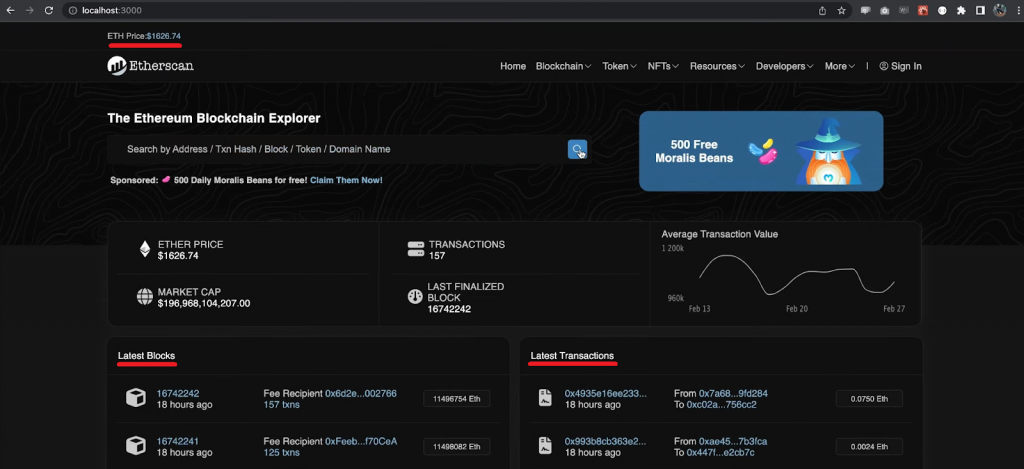
Tutorial: Create a Blockchain Explorer Backend with NodeJS and Moralis
The above screenshot exhibits our Etherscan dapp operating on “localhost: 3000”. It marks among the on-chain information that the backend must fetch. Apart from the present Ethereum worth, the most recent blocks, and the most recent transactions, our block explorer additionally permits customers to seek for transactions by the pockets handle. Additionally, our backend queries all of the associated on-chain information with the endpoints offered within the intro. Nevertheless, earlier than we present you methods to implement these snippets of code to create a blockchain explorer backend, let’s be sure you set issues up appropriately.
Backend Setup
Begin by creating a brand new venture listing. You’ll be able to comply with our lead and title it “etherscan-moralis-clone”. In actual fact, you should utilize your terminal and enter the next command to do precisely that:
mkdir etherscan-moralis-clone
Then, you must cd into that listing to create a “backend” folder with this command:
mkdir backend
Subsequent, cd into the “backend” folder and initialize a brand new NodeJS app:
npm init -y
Lastly, set up all of the required dependencies with the next command:
npm i cors moralis specific dotenv
Transferring on, open your venture in Visible Studio Code (VSC). First, deal with the “package deal.json” script and add begin below scripts like so:
“scripts”: {
“begin”: “node index.js”,
“take a look at”: “echo “Error: no take a look at specified” && exit 1″
With the above line of code added to your script, put it aside and create a brand new “.env” file:
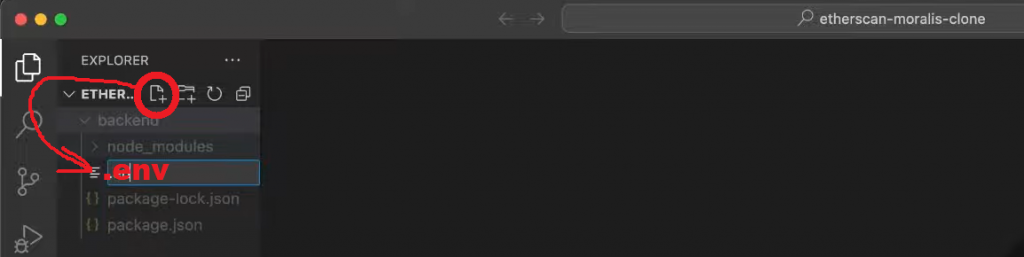
Contained in the “.env” file, create an environmental variable and name it MORALIS_API_KEY. As for this variable’s worth, you must get hold of your Web3 API key and use that worth. That is the place you must use your Moralis account. As soon as signed in, go to the “Web3 APIs” web page of your admin space and replica your API key:
Lastly, return to the “.env” file and paste the above-copied key subsequent to the MORALIS_API_KEY variable.
Coding Your Blockchain Explorer’s Backend Script
Inside your “backend” folder, create a brand new “index.js” file and first import the above-installed dependencies and decide a neighborhood port on your backend:
const specific = require(“specific”);
const app = specific();
const port = 5001;
const Moralis = require(“moralis”).default;
const cors = require(“cors”);
require(“dotenv”).config({ path: “.env” });
Subsequent, you additionally must instruct your app to make use of CORS and Specific:
app.use(cors());
app.use(specific.json());
Earlier than you truly begin implementing the Moralis Web3 Information API endpoints, make certain to additionally outline a brand new variable that shops your API key on this script:
const MORALIS_API_KEY = course of.env.MORALIS_API_KEY;
Add the Moralis.begin operate to initialize Moralis utilizing your API key. This must also be the cue to start out listening to your backend port:
Moralis.begin({
apiKey: MORALIS_API_KEY,
}).then(() => {
app.pay attention(port, () => {
console.log(`Listening for API Calls`);
});
});
With the above strains of code in place, you’re prepared to start out implementing the endpoints from the intro.
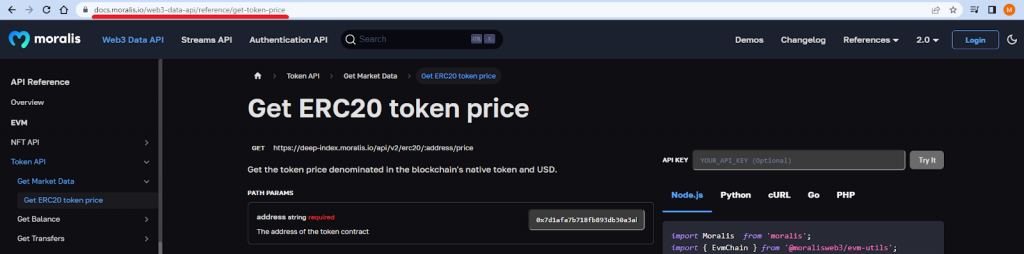
Fetch ETH Worth
The primary endpoint we’ll implement is likely one of the Moralis Token API endpoints: getTokenPrice. This endpoint fetches the token worth denominated within the blockchain’s native token and USD. In our case, we’ll use this endpoint to fetch the worth of ETH, which is Ethereum’s native coin, in USD. We have to cross two parameters: the token’s contract handle and the chain ID. So, listed here are the strains of code querying the worth of ETH:
app.get(“/getethprice”, async (req, res) => {
strive {
const response = await Moralis.EvmApi.token.getTokenPrice({
handle: “0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2”,
chain: “0x1”,
});
return res.standing(200).json(response);
} catch (e) {
console.log(`One thing went incorrect ${e}`);
return res.standing(400).json();
}
});
Wanting on the strains of code above, you possibly can see that we additionally catch potential errors and reply to them with “One thing went incorrect”.
Fetch Block Particulars
The above endpoint will allow our dapp to show the ETH worth; nonetheless, we additionally need it to show the most recent block and the most recent transactions. For that objective, we’ll use the next two Moralis endpoints: getDateToBlock and getBlock. The previous takes within the unix date in milliseconds and returns the closest block to that date. As such, it supplies us with the most recent block and a few of its particulars, together with its date, block quantity, timestamp, hash, and guardian hash. Then, we are able to use that block’s block quantity or hash as a parameter for the getBlock endpoint. In return, the latter supplies us with the small print of that block, together with the on-chain transactions. Right here’s how we make the most of the 2 endpoints in a brand new /getblockinfo endpoint:
app.get(“/getblockinfo”, async (req, res) => {
strive {
const latestBlock = await Moralis.EvmApi.block.getDateToBlock({
date: Date.now(),
chain: “0x1”,
});
let blockNrOrParentHash = latestBlock.toJSON().block;
let previousBlockInfo = [];
for (let i = 0; i < 5; i++) {
const previousBlockNrs = await Moralis.EvmApi.block.getBlock({
chain: “0x1”,
blockNumberOrHash: blockNrOrParentHash,
});
blockNrOrParentHash = previousBlockNrs.toJSON().parent_hash;
if (i == 0) {
previousBlockInfo.push({
transactions: previousBlockNrs.toJSON().transactions.map((i) => {
return {
transactionHash: i.hash,
time: i.block_timestamp,
fromAddress: i.from_address,
toAddress: i.to_address,
worth: i.worth,
};
}),
});
}
previousBlockInfo.push({
blockNumber: previousBlockNrs.toJSON().quantity,
totalTransactions: previousBlockNrs.toJSON().transaction_count,
gasUsed: previousBlockNrs.toJSON().gas_used,
miner: previousBlockNrs.toJSON().miner,
time: previousBlockNrs.toJSON().timestamp,
});
}
const response = {
latestBlock: latestBlock.toJSON().block,
previousBlockInfo,
};
return res.standing(200).json(response);
} catch (e) {
console.log(`One thing went incorrect ${e}`);
return res.standing(400).json();
}
});
Wanting on the above strains of code, you possibly can see that you just get to create a blockchain explorer that shows particulars for the most recent block and the earlier 4 blocks. That is what the above for loop is answerable for. The latter ensures that our script makes use of the Moralis.EvmApi.block.getBlock technique on the final 5 blocks. Additionally, utilizing the guardian hash (t.i., earlier block hash), our code can get hold of blocks’ particulars (transactionHash, time, fromAddress, toAddress, and worth). Lastly, it pushes these particulars to the previousBlockInfo array. Nonetheless, we additionally examine for potential errors.
Fetch Decoded Transaction Particulars
We additionally need to create a blockchain explorer backend that’s able to acquiring transaction particulars for any pockets handle. Fortuitously, Moralis affords a strong endpoint that may get hold of these particulars and decode them on our behalf. That is the place the getWalletTransactionsVerbose endpoint enters the scene.
Since we’re focusing our blockchain explorer on the Ethereum chain, our chain parameter should once more match Ethereum (0x1). The second required parameter is a pockets handle. On this case, we don’t need to use a set handle. As an alternative, we wish the endpoint to make use of the handle customers enter within the search discipline on the shopper aspect. Plus, similar to with the earlier two app.get capabilities, we additionally need to catch any potential errors. As such, these are the strains of code that get hold of decoded transactions particulars:
app.get(“/handle”, async (req, res) => {
strive {
const { question } = req;
const chain = “0x1”;
const response =
await Moralis.EvmApi.transaction.getWalletTransactionsVerbose({
handle: question.handle,
chain,
});
return res.standing(200).json(response);
} catch (e) {
console.log(`One thing went incorrect ${e}`);
return res.standing(400).json();
}
});
Be aware: If you happen to want to be taught extra about or take a look at the Moralis Web3 API endpoints, make certain to go to the API reference pages (as indicated in screenshots above every of the final three subsections). You could find the pages out there’s industry-leading Web3 documentation.
The above-outlined endpoints deal with all of the blockchain-related backend features of our Etherscan clone. As such, all that’s left to do is to create a correct frontend that neatly makes use of the above-built backend. For that objective, you get to create a NextJS app and several other JavaScript parts. If you happen to want to comply with our lead and create a blockchain explorer frontend as properly, make certain to make use of the video a the highest of the article, beginning at 20:49. That will help you resolve if our frontend is worthy of your time, make certain to take a look at a demo beneath.
Our Etherscan Clone Blockchain Explorer Demo
The next screenshot exhibits all the small print of our blockchain explorer dapp:
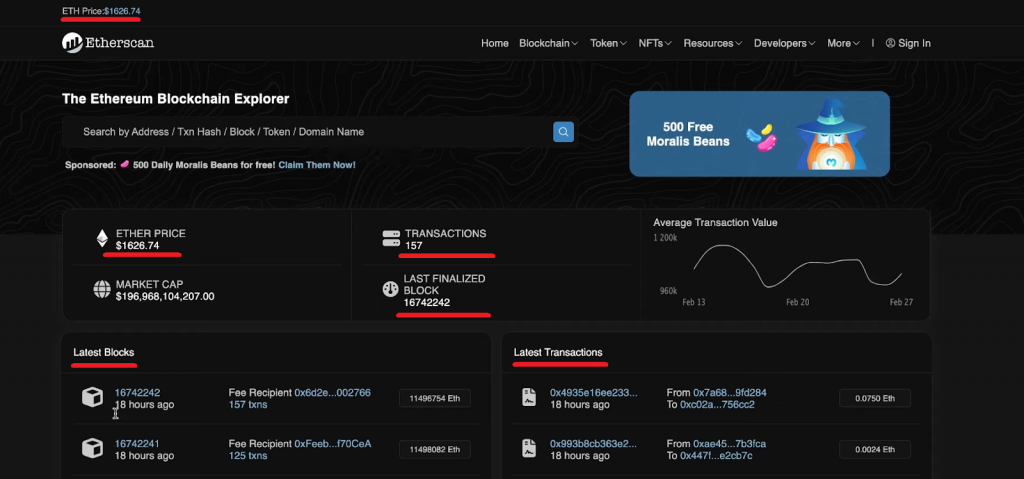
All the underlined particulars within the above screenshots are fetched by the highly effective endpoints from Moralis. These embody the ETH worth, newest transactions, block variety of the final finalized block, particulars of the final 5 blocks, and particulars of the most recent transactions. In fact, there’s additionally a search field, which is the core of our blockchain explorer. That is the place customers can paste any pockets handle to discover all its transactions:

Our frontend shows all of the transactions and their particulars in a neat desk that follows Etherscan’s appears:
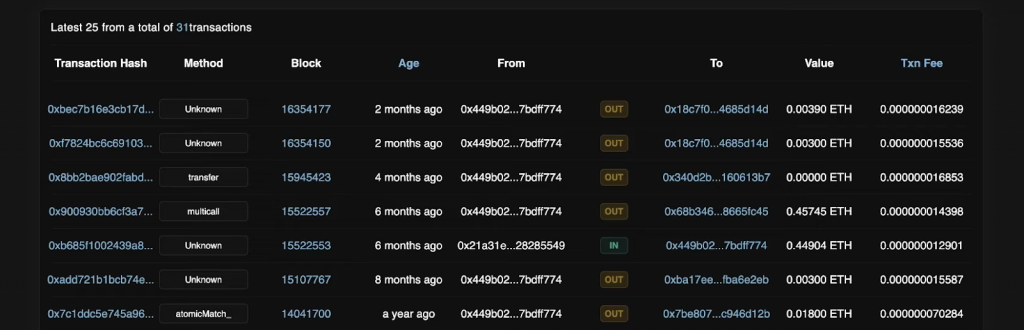
If you happen to want to take our instance blockchain explorer dapp for a spin however don’t want to code it from scratch, merely clone the code that awaits you on our “etherscan-clone” GitHub repo web page. Apart from all of the frontend scripts, that is additionally the place yow will discover the whole “index.js” backend script coated above.
Be aware: In case you’re questioning what “Moralis Beans” are, make certain to take a look at the main specialised blockchain explorer: Moralis Cash. That is the place yow will discover true crypto alpha tokens and accumulate 500 Moralis Beans every day.
What’s a Blockchain Explorer?
A blockchain explorer is a particular type of decentralized software (dapp) enabling you to discover the publicly out there on-chain information. With a correct blockchain explorer, you possibly can view token particulars, transactions, handle balances, and extra.
You in all probability know that there are numerous programmable blockchains up and operating, and every blockchain has its personal official blockchain explorer. Main examples embody Etherscan for Ethereum, PolygonScan for Polygon, BscScan for BNB Chain, SnowTrace for Avalanche, FTMScan for Fantom, and so forth. Apart from Ethereum and EVM-compatible chains, there are additionally blockchain explorers for non-EVM-compatible chains. An incredible instance of that’s the Solana explorer Solscan.
Since all chains have their official blockchain explorers, why do you have to create a blockchain explorer your self? Effectively, the 2 fundamental causes are simplification and consumer retention. If you happen to’ve used any of the aforementioned block explorers, you already know that their UX expertise will not be nice, particularly not for crypto novices. Thus, it is smart to create blockchain explorers with nice UIs that simplify the expertise. Maybe you possibly can obtain that by focusing solely on particular options. Furthermore, by including a user-friendly blockchain explorer to your dapps, you possibly can make sure that your customers keep round whereas checking if their transactions went by means of.
How one can Create a Blockchain Explorer – Abstract
In in the present day’s article, you had an opportunity to comply with our lead and create a blockchain explorer backend. If you happen to determined to stay round and full our tutorial, you created a NodeJS dapp powered by an “index.js” script. Inside that script, you carried out 4 highly effective Moralis Web3 Information API endpoints. You additionally discovered methods to get hold of your Web3 API key and retailer it in a “.env” file. Moreover, you had a possibility to get impressed by our demo to create a frontend on your blockchain explorer. Now, when you determined to create a NextJS app that handles the frontend, you had two choices:
You may construct it from scratch following the video tutorial on the prime of this text. Or, you could possibly’ve merely cloned our frontend script from our GitHub repository.
All in all, you now know methods to create a quite complicated dapp utilizing JavaScript and Moralis. As such, you’re able to deal with other forms of dapps.
When you’ve got your personal dapp concepts, simply dive into the Moralis docs and begin BUIDLing. Nevertheless, when you want inspiration, motivation, and/or need to be taught extra about Web3 improvement, go to the Moralis YouTube channel and weblog. That is the place yow will discover quite a few glorious tutorials and blockchain improvement subjects. Among the newest articles dive into the Solana devnet, Web3 market improvement, constructing a Polygon portfolio tracker, exploring the main Goerli faucet, and way more. In case you want help with the technical features of scaling your dapps, make certain to succeed in out to our gross sales workforce!
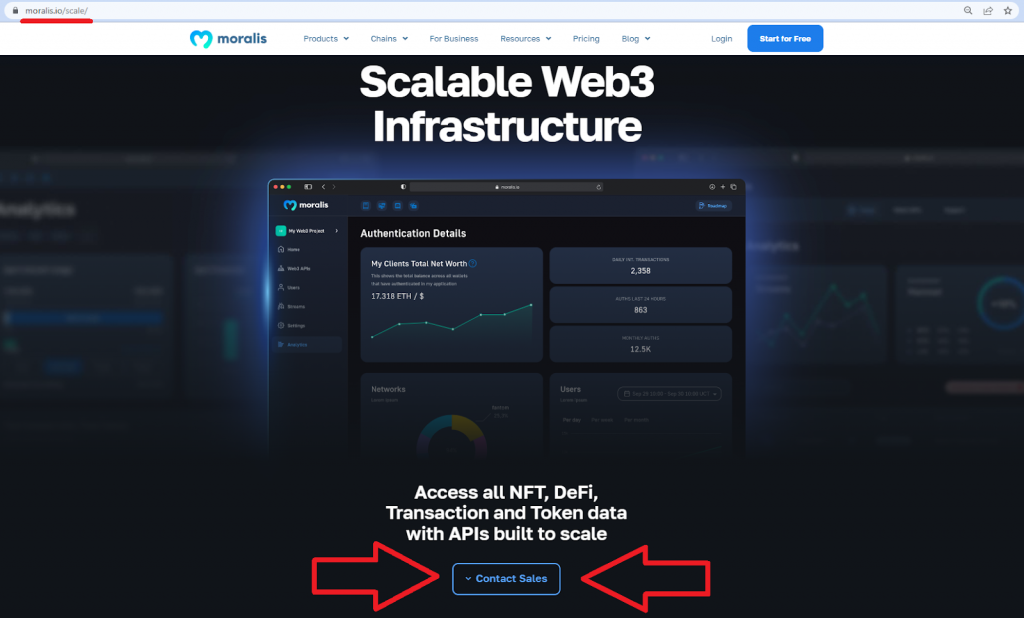